JavaScript is still one of the most popular programming languages used for software development today. If you are familiar with JavaScript, it is likely you have also heard of TypeScript.
To understand what Typescript is and why it has been created, let’s briefly look at JavaScript, as both languages are closely related to each other.
Table of Contents
JavaScript
JavaScript is a dynamically typed programming language, which means that it is a very flexible programming language where the types of a variable will not be defined until the program is executed. Therefore, developers do not need to define variable types when creating them. Many developers, expecially newcomers, love this aspect because it makes learning and writing code easier and faster. In general, whatever you write, JavaScript will try to find a way to execute it.
So for example, in a JavaScript code:
var random_variable;
var this_is_a_text = "true";
this_is_a_text = 3;
You can see that the same variable called “this_is_a_text” first stores a text and then later stores a number. Additionally, when the variable is created, there is no indication of what it should store.
That is quite different from other programming languages like Java or C++ which are statically typed programming languages. In those languages, you must define the type of everything beforehand. Furthermore, before the execution of the program, many checks are performed to ensure that the code you wrote makes sense. If a mistake is found, such as trying to store a number in a variable that was intended to store only text, the code will not execute until the problem is resolved.
These “checks” performed by programming languages like Java und C++ are beneficial because they help ensure better code quality. However, this requirement of the programming language also necessitates that programmers plan their code structure more thoroughly. This often means that developers require more time to write the code, especially in the beginning when they are learning a new language.
So, now we understand that JavaScript is a dynamically typed programming language, which provides a lot of flexibility to developers. It makes writing code faster and easier, particularly for short code snippets designed to solve specific tasks. However, many developers eventually realize that this flexibility can become a significant problem as the codebase and the number of developers involved in the project grow.
The scalability problem with JavaScript
Let’s consider a common scenario: you have a large development team working on an application with hundreds of interdependent files. Any change made to one file could potentially impact the behavior of many other files and potentially break the application if an error is introduced.
To ensure that new changes do not introduce errors, developers typically write automated tests to verify that the code functions as expected. They also rely on code reviews from other developers to ensure the changes are acceptable. These practices are fundamental and help ensure the stability of the codebase when it is large and involves multiple developers. However, the effort required to check the relationships between all the dependent files through tests and code reviews can become substantial as the codebase and development team grow.
Now, consider the same scenario mentioned earlier, but from the perspective of a new developer who has just joined the company. You are tasked with solving a small problem in a specific part of the codebase. However, understanding the contents of each variable and the expected data structure within various parts of the code can be time-consuming. It may require analyzing the code extensively and even manually executing the application to grasp something as simple as what should be contained within a single variable.
Hence, there must be a better way in JavaScript automatically validate code correctness and make the data structure explicit, especially for new or unfamiliar developers.
Introducing TypeScript!
TypeScript
TypeScript is a programming language similar to JavaScript, but it is considered a superset of JavaScript. This means that TypeScript encompasses all the main features of JavaScript while also introducing additional features that are exclusive to TypeScript. Therefore, any code written in JavaScript is also valid TypeScript code, but the reverse is not necessarily true.
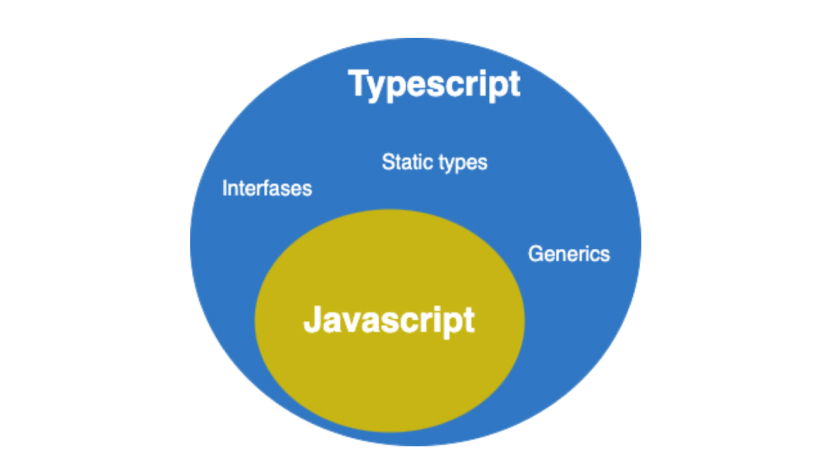
The main feature that TypeScript adds on top of JavaScript is the capability to add explicit types for the variables on the code. As they define on their site: TypeScript is JavaScript with syntax for types.
Now in TypeScript, a developer can write this:
type MyType = {
name: string;
Value: number;
}
var random_variable:MyType;
var this_is_a_text:string = "true";
That makes the code way clearer for any developer trying to understand what each variable is expected to have inside. On top of that, if any changes are made TypeScript will check if they make sense, so if a developer tries to add a line like this one:
this_is_a_text = 10
TypeScript will give an error saying that this is not possible, because the variable “this_is_a_text” should only store text not numbers.
Of course, in our example, that looks obvious, but when there are multiple files involved with many dependencies between them, it is not so easy to realize that. But additionally, the TypeScript checks help a lot in finding errors caused by a major enemy of developers: typos. Just look at the example provided by TypeScript:
const announcement = "Hello World!";
// How quickly can you spot the typos?
announcement.toLocaleLowercase();
announcement.toLocalLowerCase();
// We probably meant to write this...
announcement.toLocaleLowerCase();
A small difference in the code, such as a lowercase letter instead of an uppercase letter, can easily go unnoticed but can cause the code to fail when executed. TypeScript can detect such errors and provide warnings to the developer.
Advantages of TypeScript
Now that you have seen TypeScript in action and understood what it adds to JavaScript, let’s explore the specific advantages that these technologies offer in large projects.
Improves readability
As demonstrated in the practical examples above. TypeScript enhances code comprehension by allowing variables to be explicitly typed. Developers don’t need to execute the code or analyze multiple lines to understand the potential content of each variable or how the data should be structured. Everything is explicitly describes alongside the code, resembling a statically typed programming language.
With improved readability, developers can update the code more confidently without introducing new errors. Additionally, debugging becomes easier as the source of existing errors can be identified more efficiently.
Scalability and stability
Through automatic type checks and enhanced readability, TypeScript contributes to code scalability and stability. Even in projects with numerous interdependent files, TypeScript ensures that new updates maintain the correct typing. Consequently, developers can work more seamlessly across different parts of the system.
Two notable examples, showcased on the TypeScript website, are Slack and Airbnb. Airbnb reported a 38% reduction in bugs introduced to their codebase after adopting TypeScript.
Compatibility with JavaScript
TypeScript is highly compatible with JavaScript. Your regular JavaScript code remains valid TypeScript code, and any code written in TypeScript will be transformed into JavaScript before execution, ensuring compatibility with all browsers.
TypeScript takes care of the transformation process, converting the code into valid JavaScript that can be executed by browsers without any issues.
Furthermore, thanks to this transformation, TypeScript allows you to leverage the latest versions of JavaScript without worrying about browser compatibility. TypeScript ensures that the resulting JavaScript code only contains features supported by the target browsers. For advanced users, there is even the option to configure this process for better control.
Gradually adoption
Due to the excellent compatibility between TypeScript and JavaScript, the adoption of TypeScript can be done gradually and incrementally. You can start creating new files in TypeScript while leaving existing in files in JavaScript. Over time, your team can gradually convert individual JavaScript files to utilize TypeScript features.
There is no need for your team to halt application development to migrate the entire system. TypeScript itself recommends a gradual transition approach.
Community and documentation
The TypeScript community is growing rapidly each day. In the StackOverflow Developer Survey 2022, TypeScript ranked as the fourth most loved technology and came in a very close third, just behind the second most wanted technology.
If your team encounters any issues with TypeScript, it is highly likely that you can easily find solutions in the extensive documentation or by seeking help from the community.
Integration with Frameworks
As TypeScript continues to gain popularity, many widely adopted code editors and frameworks have excellent integration with TypeScript. This enhances developer productivity and improves programming efficiency.
Code editors such as VSCode (Visual Studio Code) provide native TypeScript support or offer plugins that leverage TypeScript. These editors can display variable type automatically when hovering over them, as well as highlight TypeScript errors in real-time while code is being written.
What TypeScript is not
Now that we understand what TypeScript is, it’s important to note what it is not. TypeScript is a language that assists developers in writing better JavaScript code primarily by adding static types that are automatically checked before code execution. However, since TypeScript is transformed into JavaScript before execution (as browsers can only execute JavaScript), there are no checks performed during runtime.
For instance, in TypeScript code, you can define the expected type of data returned from an external service , allowing developers to better understand the code and make appropriate updates. However, if the service unexpectedly returns different data during code execution, such as due to an update on their end, no errors will be thrown.
TypeScript’s type checks occur during development, aiming to improve developer efficiency and minimize errors. But TypeScript itself is not executed in the end. Therefore, no checks are performed during runtime. The code that ultimately executes is JavaScript.
Costs of using TypeScript
We’ve highlighted the incredible benefits of TypeScript and why you may want to adopt it for your project. But what are the associated costs, particularly when transitioning an existing JavaScript project to TypeScript?
Learning the language
The initial cost lies in training your team to work with TypeScript if they have no prior experience. Fortunately, since TypeScript is closely related to JavaScript, the learning curve is relatively small. It’s not a drastic change for JavaScript developers to start using TypeScript. Moreover, you can gradually introduce TypeScript by initially adding special comments to your JavaScript code until you transition to the complete TypeScript syntax.
The first adoption
Once your team becomes more comfortable with TypeScript, the next cost is updating the entire codebase to use the new language. This cost can be significant for large an complex applications, as it involves modifying the entire codebase. However, as we discussed earlier, TypeScript adoption should be done gradually. Even though the cost may be high, it can be spread over time. You can begin by changing one file at a time, gradually reaping the benefits of the language without burdening the company with the cost and risk of a full codebase update.
Overhead
Lastly, there is a constant cost associated with the presence of types in the code. With TypeScript, developers need to be more diligent in typing and better plan the system before development. They also need to handle type adjustments when updates are made to maintain consistency and functionality. This naturally adds an overhead. Previously, developers only needed to change the code itself, but now they also have to consider the types involved.
However, this overhead diminishes as the team becomes more proficient in TypeScript. Moreover, it is minimal compared to the cost reductions gained from the benefits of TypeScript. With a more stable codebase and fewer errors in the application, the cost reduction in maintenance far outweighs the added overhead.
Advantages Of TypeScript - Conclusion
TypeScript is indeed a powerful language that enhances scalability, readability, and error reduction in JavaScripts projects. It can effectively reduce maintenance costs and enhance the development experience for JavaScripts projects, especially those that are growing in complexity and continuously evolving. TypeScript’s popularity and growth in the software development industry make it a valuable asset for expanding and improving JavaScript projects.